A. Compilation: To create and run Java programs in your computer, you have to download and install "Java" - what have you downloaded and installed? why a PC and a MacBook have to download different packages?
Answers:
1. The first part of the package is JVM. JVM communicates with OS and runs Java "byte code". Because a PC and a MacBook run different OS, their JVMs are different. That's why you have to download different packages for PC and Mac.
2. The second part of the package is a Compiler. The compiler turns your Java programs(.java files) into "byte code"(.class files) so they can run in JVM. The compiler works behind the scene as you edit your programs(source code), the process of turning your source code into byte code is called compilation.
3. The third part of the package is Java Standard Library, which has many built-in useful classes and methods such as String, Scanner or Math.sqrt() etc.
B. Compile-time Errors and Run-time Exceptions
The compiler may detect errors, mistakes or ambiguous definitions while processing the source code. It will report these errors to programers so they can correct. These are compile-time errors. Examples are type mismatching, undefined variables, invalid method call etc. If these errors are not correct, compiler will not be able to turn the source code into byte code, so the program won't run at all.
If a program has no compile-time errors, compiler will run the code. During the execution of code, there might be some exceptions such as dividing by 0, wrong input format, array or string index out of bound etc. These errors happen while the program is running, are called run-time exceptions.
Checked and Unchecked Exceptions: The checked and unchecked exceptions both happen at run time, while the program is in execution. If the program specifies clearly what to do when an exception happens, (i.e. how to handle this exception) it is a checked exception. For unchecked exceptions, nothing is specified so it is up to JVM to give some error messages if the exception rises.
C. Packages: Now that we understand the underline platform which supports a Java program, find out:
How are Java programs organized into packages? What is the default package?
When you program, how to use standard classes provided in Java library?
What's the structure of a Java class?
Answers:
1. Java standard library classes are organized in packages and sub packages such as java. util. *, or java.lang.*. Here the top level package is "java" while "util" and "lang" are two sub packages. There are many classes in each sub package.
2. To use the standard library classes, you need to import the sub packages or classes in your class. By default, java.lang.* is always imported to any custom class.
3. A java class is put in "default" package if you don't create one. This default package is under your "project" folder in Eclipse or Codiva.
D. Publicity Modifiers: Any Java class or class members can have a publicity modifier: public, protected, private, or default (not specified). What does these modifier mean?
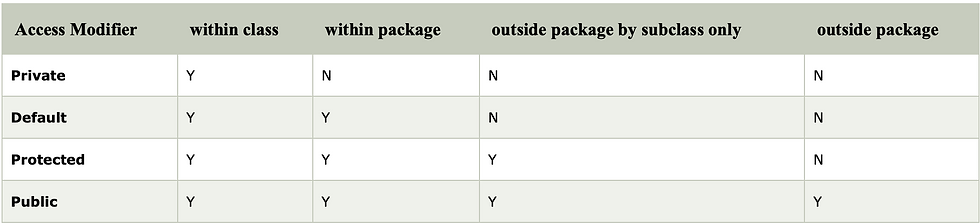
E. Java input/output
Scanner class is not required by AP CSA.
System.out.printf is not required by AP CSA, only System.out.print and System.out.println.
What will "System.out.println(4+6+"lb");" print out? How about "System.out.println("$"+4+6);"?
What are escape sequences?
Answer: System.out.println("Hello World!"); will never print out double quote ". If you need to print " as part of the output, you can use escape sequences. An escape sequence is a backslash \ followed by another character, Java use these to print out some special characters.
For instance, \" prints ", \n prints a new line and \\ prints backslash \ itself.
F. HW
Multiple Choice Questions: #1-8 of Chapter 2(Barron's). Please check your answers AFTER you solve the problems. Post here if you have more questions about the correct answers.
C2:
There are two ways you can pass a variable primitive vs reference.
Passing by refernece is used because objects cant have one storage unit they need multiple which is called an area.
Passing by reference is used because objects cant have one storage unit they need multiple which is called an area.
ex: Cost c = new Cost(2.5); // have to follow the values that can is legal given in the constructor is a refernece
C3: Type casting in java refers to converting a value into another. ex: double --> int
Syntax: double d = 2;
int x = (int)d;
Up-casting is the conversion (casting) of a subclass or an object to a superclass.
Down-casting is the opposite (super to sub).
C1. How to declare a class? a variable? a method?
Classes are declared at the top of the code, with a public or private keyword. Variables require a data type, and an initialization value, that has the correct data type. Methods require a name with parentheses, data type, and a public or private keyword.
Class ex: public Dog {
Variable ex: int age = 7;
Method ex: public void Name() {
B2. When you program, how to use standard classes provided in Java library?
You can import them by typing import and the class's name. For example, if you want to import scanner, you can type import java.util.Scanner or you can type import java.util.* to import all classes in util.
B3: Access modifier (private or public class) + the class keyword + Name of the class + opening curly brace
+ instance variables + many kinds of methods
+ closing curly brace.
A1: JVM is the Java Virtual Machine. To compile the c ode, a compiler is used. It turns your code into "machine language," and then the JVM reads the class file and turns it into binary, so that your computer can run your code.
D3: This will print out 10lb because the first two are integers. The second one will print $46 because the first character is a string. If you wanted to do $10, add parentheses around the 4 and 6.
B1:
Similar/related classes are organized into one package.
The default package is the Object package, which contains int ,double, lang, String....
A3:
1. A compile time error occurs during compilation of the program from a .java to a .class file. This is typically a design flaw in the program itself.
2. A run time error occurs when the program is executed after compilation.This is usually a bad value that breaks the program.
3. An unchecked error is the error that the program throws when it is running and can be caused by bad data. These are checked at runtime and prevent successful execution.
4. A checked error is the error that the program throws when it finds an issue in the program structure. These errors are checked at compile time and prevent successful compilation.
D1 & D2:
For input and output in the scope of AP CS A, we only need to know:
System.out.print() and
System.out.println().